The best way to meet wxBasic is to look at some simple lines of code.
To write a simple "Hello, World" on the screen, this is enough:
wxMessageBox( "Hello, World" )
The samples:
Minimal sample
Even a more or less complete Windows application with a frame, menu items and a status bar is very simple and quick to write.
The "Minimal sample" is taken from the samples of wxWidgets translated to wxBasic.
The source code
' minimal wxBasic sample ' based on code by Julian Smart option explicit ' create the main application window dim frame as wxFrame = new wxFrame( Nothing, wxID_ANY, "Minimal wxBasic App" ) ' create a "File" menu and append an item dim mFile = new wxMenu() mFile.Append( wxID_EXIT, "E&xit\tAlt-X", "Quit this program" ) ' create an "About" menu and append an item dim mHelp = new wxMenu() mHelp.Append( wxID_ABOUT, "&About...\tCtrl-A", "Show about dialog" ) ' now append the freshly created menu to the menu bar... dim menuBar = new wxMenuBar() menuBar.Append(mFile, "&File") menuBar.Append(mHelp, "&Help") ' ... and attach this menu bar to the frame frame.SetMenuBar(menuBar) ' create a status bar frame.CreateStatusBar(2) frame.SetStatusText("Welcome to wxBasic!") ' callback for the Quit menu option function OnQuit( event ) handles frame.menuSelected(wxID_EXIT) ' TRUE is to force the frame to close frame.Close(True) end function ' callback for the About menu option function OnAbout( event ) handles frame.menuSelected(wxID_ABOUT) dim msg = "This is the \"About\" dialog of the Minimal sample.\n" & "Welcome to wxBasic!" wxMessageBox( msg, "About Minimal", wxOK + wxICON_INFORMATION, frame ) end function ' frames, unlike simple controls, are not shown when created frame.Show(True)
SVG View
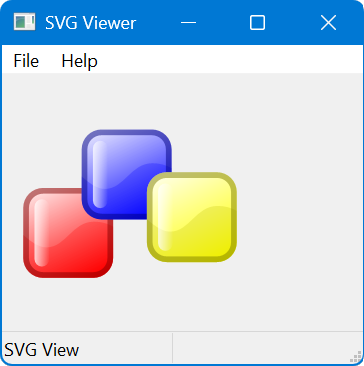
With the new wxBitmapBundle class svg-files can be used, also embedded.
wxWebView
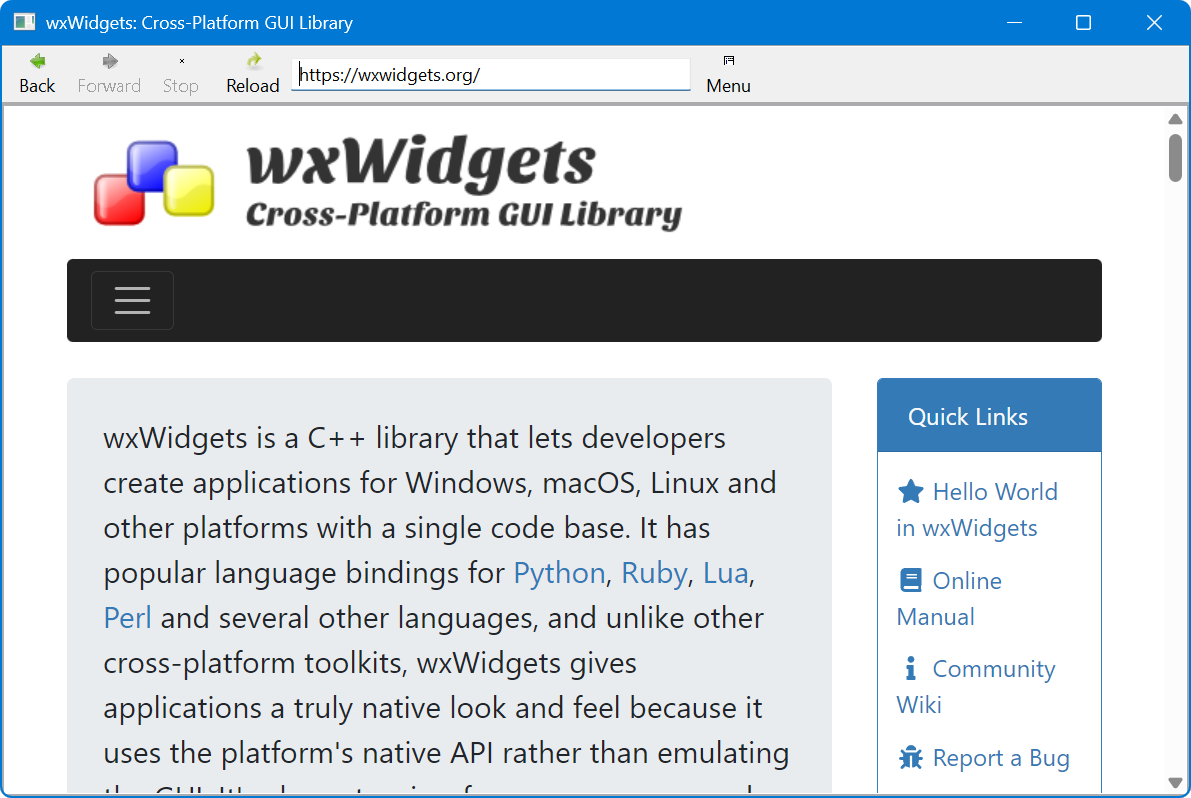
wxWebView may be used to render web (HTML / CSS / javascript) documents.
It is designed to allow the creation of multiple backends for each port, although currently just one is available. Each backend is actually a full rendering engine, Internet Explorer or Edge on MSW and WebKit on macOS and GTK. This allows the correct viewing of complex pages with JavaScript and CSS.
wxRibbonBar
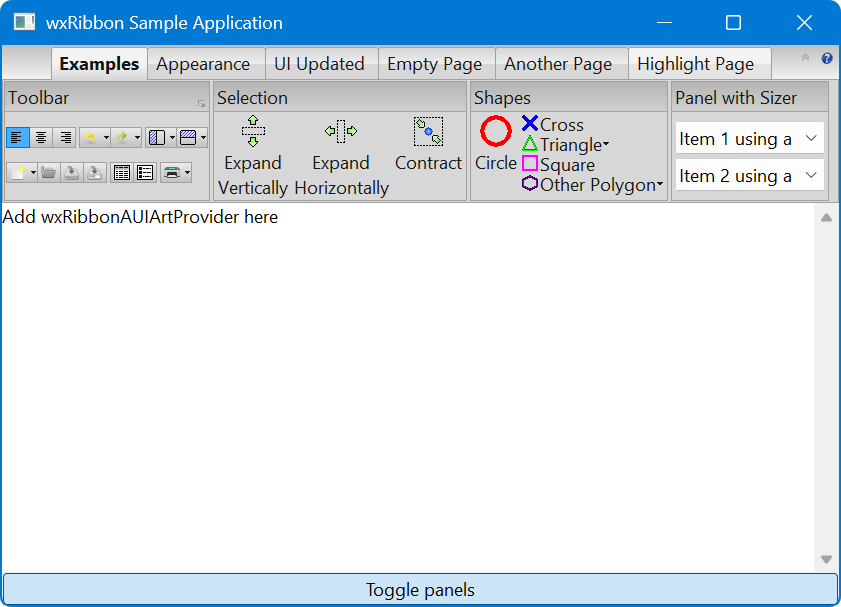
The RibbonBar sample demonstrate the possibility to use a ribbon-bar beside or instead of classical menu-bar and tool-bar.
wxRibbonBar serves as a tabbed container for wxRibbonPage - a ribbon user interface typically has a ribbon bar, which contains one or more wxRibbonPages, which in turn each contain one or more wxRibbonPanels, which in turn contain controls.
wxRichTextCtrl
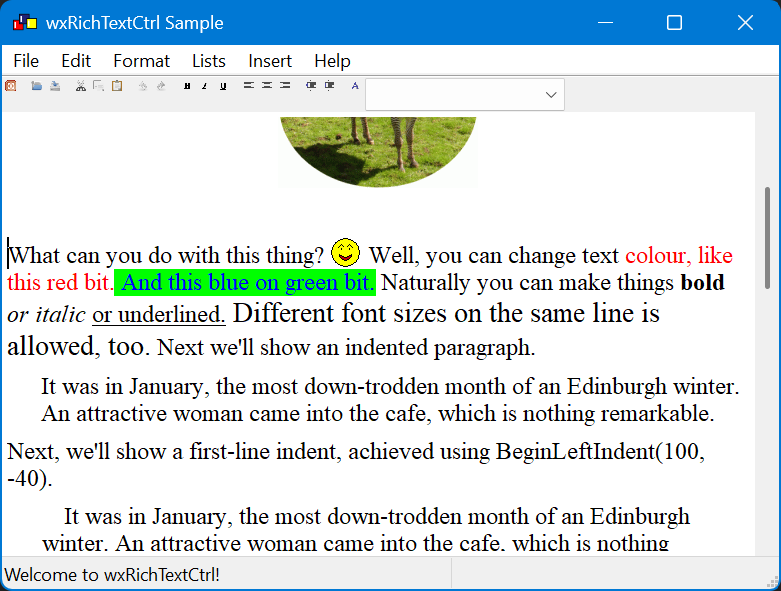
The RibbonBar sample demonstrate the possibility to use a ribbon-bar beside or instead of classical menu-bar and tool-bar.
wxRichTextCtrl provides a generic, ground-up implementation of a text control capable of showing multiple styles and images.
wxEditor sample (by Dirk Noak)
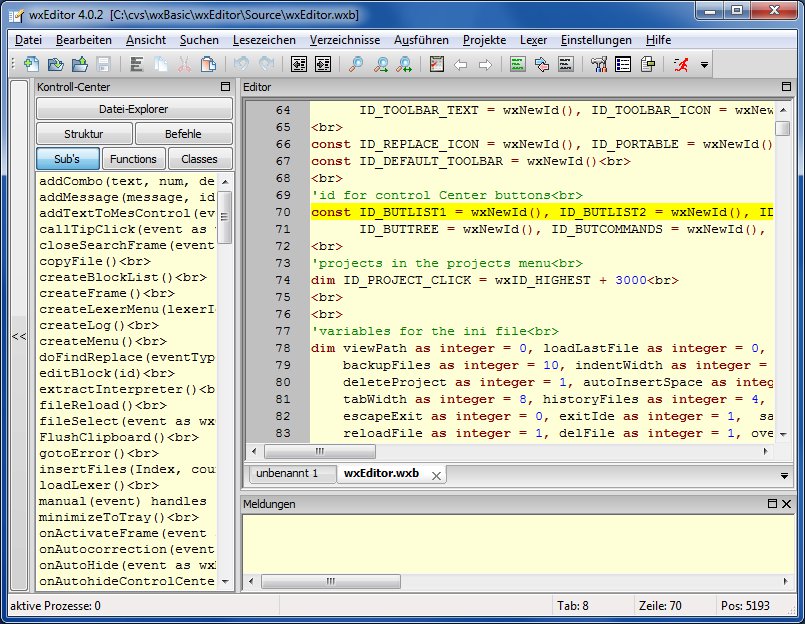
A full featured development environment for wxBasic in Beta stage. Is is based on David Cuny's IDE sample.
Calculator sample
This is a complete new calculator that demonstrate some of the new features: XRC support, AcceleratorTable support, ...
Media Player sample
This is a new sample that demonstrate the wxMediaCtrl control.
It was originaly written by John Labenski in wxLua and translated to wxBasic in a few minutes.
wxAui sample
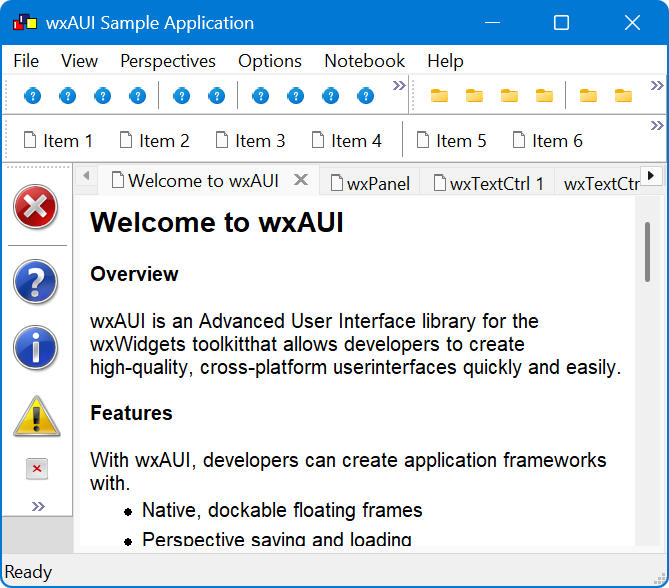
This sample demonstrate the use of wxAui classes to manage panes.
wxAUI stands for Advanced User Interface.
It aims to give the user a cutting edge interface with floatable windows, and a user-customizable layout. The original wxAUI sources have kindly been made available under the wxWindows licence by Kirix Corp. and they have since then been integrated into wxWidgets and further improved.